When scraping websites with login pages, a challenge arises with passing through the login page to gather the data you need. However, there is a way to bypass the login page and get straight to scraping. This guide will introduce the challenges associated with scraping websites with login pages, setting up an environment, analyzing the login mechanism, and how to create the Python script necessary to scrape through a login page. This guide can only assist with bypassing the login page to a website where a user already has the login information on it. Bypassing a login page without having any login credentials is unethical and could be illegal.
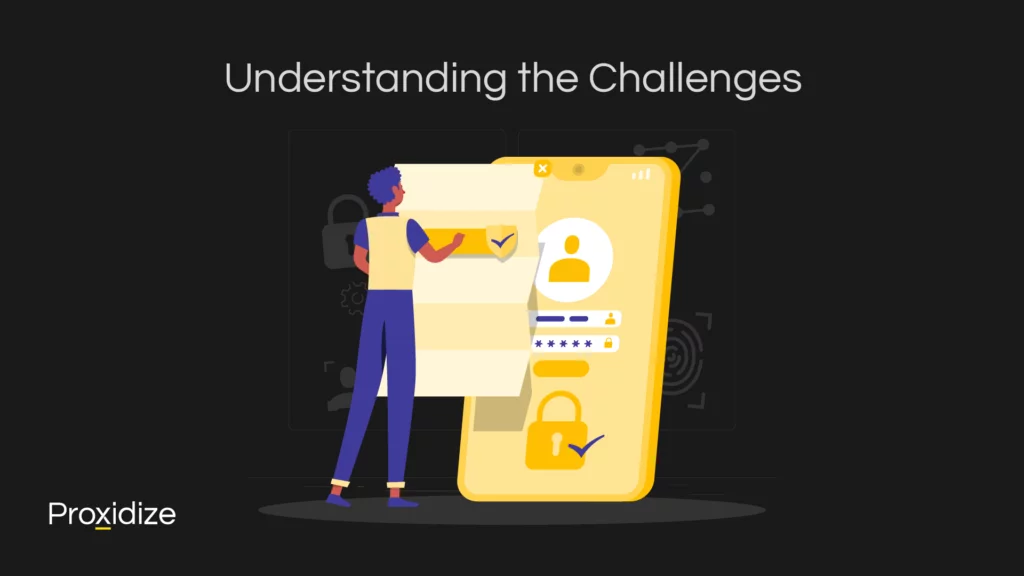
Understanding the Challenges
Scraping websites that require a login is more complex than scraping public pages as it usually involves additional metrics that typical scraping does not include. This involves maintaining an active session, managing cookies, and dealing with CAPTCHAs.
Scraping public pages involves sending a GET request to retrieve the HTML content of the pages. With authenticated pages, it is necessary to login to the website by submitting the credentials first, just like a normal user would when accessing their account. This process involves submitting a POST request with form data such as a username, password, and security tokens. One must also ensure that a session is maintained after login so that authenticated requests can be made to access protected resources.
Once logged in, every request must be authenticated through session cookies or tokens. This session management adds a layer of complexity as improper handling can result in denied access. Websites with login requirements use sessions and cookies to keep track of authenticated users. Scrapers need to maintain an active session throughout their process which is achieved by using requests.Session() object in Python. This is presented in visual details further down the article. This will store cookies and session data across multiple requests.
Cookies need to be saved and sent along with each subsequent request so that sessions remain authenticated. Without cookies, the server may treat each request as unauthenticated and deny access. Some websites use Cross-Site Request Forgery (CSRF) protection. This includes hidden tokens in forms that must be submitted along with the login details. If the token is not sent or is incorrect, the server will reject the request.
Finally, CAPTCHAs pose a significant roadblock when it comes to scraping in general. There are a few methods that could circumvent CAPTCHA including integrating CAPTCHA-solving services or avoiding websites that use advanced CAPTCHA methods.
Some websites implement more advanced detection techniques. One of the ways this could be bypassed is by using mobile proxies to hide the IP address and make the traffic appear as if it is coming from somewhere else. This could be strengthened by using rotating proxies as they would intensify the anti-detection practices by allowing users to access ever-changing IPs.
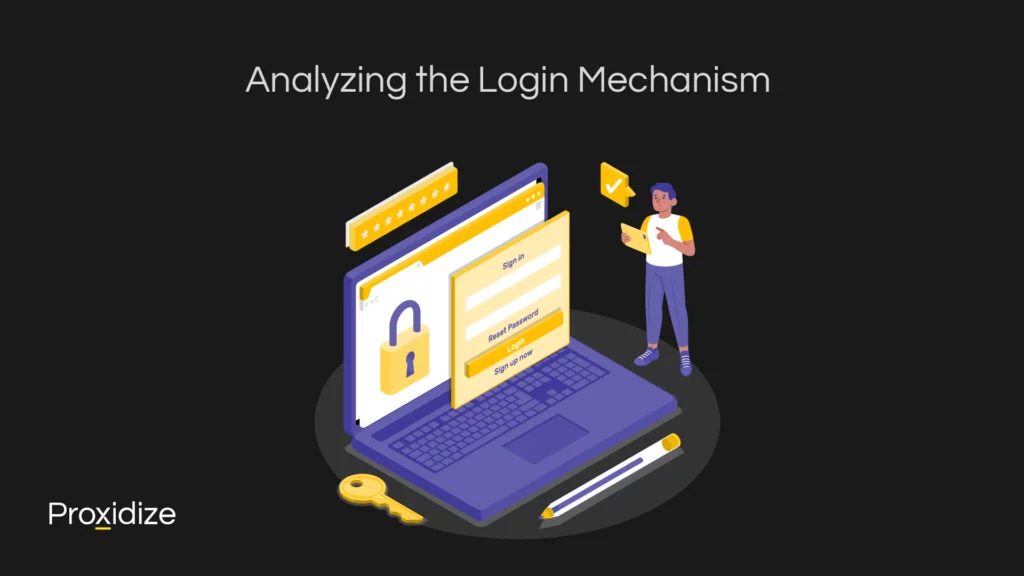
Analyzing the Login Mechanism
Scraping websites with a login page requires understanding how a website’s login process works. To do this, a user must inspect the login form, identify key fields, and observe how form submission happens. This can be done by following these steps:
Inspect the Login Form on the Website: Once on the login page of the desired website, open the developer tools by right-clicking on the page and selecting “Inspect” or by pressing the hotkey Ctrl+Shift+I. Look at the HTML structure of the form to find the input elements for the login. This includes anything from Username Field (name=username/id=username), Password Field (name=password), and CSRF Tokens which must be included in the form submission to successfully authenticate the user.
Understand Form Submissions: Check if the form uses a POST method for submission. The form’s “action” attribute specifies the URL that the data is sent to. The data can typically include the username, password, and other hidden fields such as the CSRF token and session identifiers.
Use Browser Developer Tools to Monitor Network Requests During Login: While developer tools are open, navigate to the “Network” tab and submit the login form with test credentials. Look at the network requests that are made during the form submission and locate the request corresponding to the login attempt which is usually labeled as POST. Click on the request to see the details which include the headers, form data, and response. This information tells the user how the server handles authentication and what data needs to be sent for a successful login.
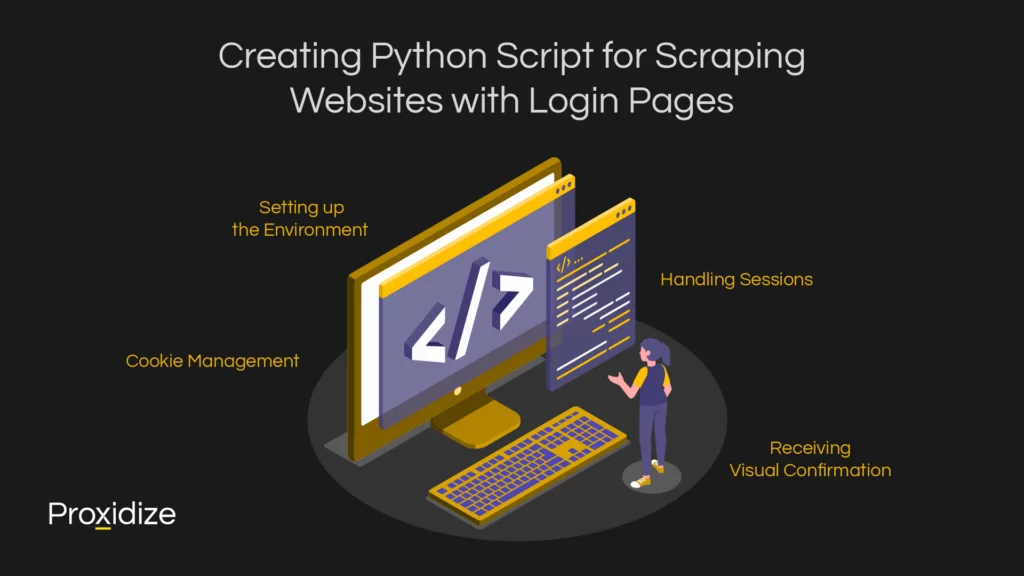
Creating Python Script for Scraping Websites with Login Pages
Setting up the Environment
The first and most crucial step in any web scraping project is to set up the core environment. For many web scraping experts, this section can be skipped as they most probably know how to do this. For everyone else, let us walk through what this means and how to set up the environment.
Setting up an environment involves explaining what tools your script will utilize. Conceptually, it is like the tool box you fill when you want to build something. Without a hammer, you would not be able to do much. The environment differs depending on your specific task or project. For scraping websites with login pages using Python, the two main “tools” necessary for this task are the Requests library and Beautiful Soup.
Requests is used in handling HTTP requests that allow you to send data to servers, maintain sessions, and manage cookies easily. It allows you to login to websites by sending POST requests and accessing authenticated pages within the same session. BeautifulSoup is used for parsing and extracting data from the content retrieved by requests. It navigates, searches, and modifies HTML documents which makes it perfect for getting data from web pages faster and more efficiently. Together, requests and BeautifulSoup provide a straightforward approach for logging in and extracting data from websites.
In the terminal of your integrated development environment (IDE), enter the following commands:
pip install requests
pip install beautifulsoup4
Once that is done, you are ready to start scraping websites with login pages.
Handling Sessions
# Create a session object to maintain cookies and headers across requests
session = requests.Session()
# Send a POST request to log in using the session
response = session.post(login_url, data=payload)
# Access another page using the same session
protected_page_url = "https://www.example.com/protected-page"
protected_response = session.get(protected_page_url)
Cookie Management
# check if the request was successful
if response.status_code == 200:
print("Login successful!")
# Display cookies received after login
print("Cookies after login:")
print(session.cookies.get_dict())
else:
print(f"Login failed with status code: {response.status_code}")
If you already have cookies for a website but do not have the login information, this can easily be bypassed with the following script:
import requests
from bs4 import BeautifulSoup
# Create a requests session
session = requests.Session()
# Example saved cookies (replace these with your actual cookies)
saved_cookies = {
"session_id": "your_session_id_value",
"auth_token": "your_auth_token_value"
}
# Load cookies into the session
for name, value in saved_cookies.items():
session.cookies.set(name, value)
# Use the session to access a protected page
protected_page_url = "https://www.scrapingcourse.com/dashboard"
response = session.get(protected_page_url)
# Parse the response using BeautifulSoup
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
page_title = soup.title.string if soup.title else "No title found"
print(f"Page title: {page_title}")
else:
print(f"Failed to access the protected page: {response.status_code}")
print(response.text) # For debugging
Full Script for Scraping Websites with Login Pages
For the script, we will be using the website https://www.scrapingcourse.com/login as an example. With everything compiled, the script should look like this:
import requests
from bs4 import BeautifulSoup
# Create a session to persist cookies and headers
session = requests.Session()
# the URL of the login page
login_url = "https://www.scrapingcourse.com/login"
# the payload with your login credentials
payload = {
"email": "[email protected]",
"password": "password",
}
# send the POST request to login using the session
response = session.post(login_url, data=payload)
# check if the request was successful
if response.status_code == 200:
print("Login successful!")
else:
print(f"Login failed with status code: {response.status_code}")
# access another page after login, maintaining the session
protected_page_url = "https://www.scrapingcourse.com/protected-page"
protected_response = session.get(protected_page_url)
# parse the protected page content using BeautifulSoup
soup = BeautifulSoup(protected_response.text, "html.parser")
# find the page title
page_title = soup.title.string if soup.title else "No title found"
print(f"Page title: {page_title}")
# Example of extracting data from the protected page
data = soup.find('div', class_='data-class') # Adjust selector based on your needs
if data:
print(f"Extracted data: {data.text}")
else:
print("No data found with the specified tag/class.")
Inputting the script above within your code will allow you to bypass the login page when scraping a website. This will save time when scraping a page such as a social media site or any other website that requires a login. It must be stated that this code alone will not start scraping a website, this will simply pass through the login page. To learn how to write code that will scrape websites, we have written an article detailing how to write a Python script for web scraping.
Receiving Visual Confirmation
The code provided above works for getting past the login page successfully. However, you might want confirmation that the script is working. To do this, you would need to introduce Selenium into the mix. To do this, install the Selenium package into your terminal by using:
pip install selenium
After that is done, you would need to add a few lines of code to inform your script that you wish to see the browser pop up. The fully updated script should look like this:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
import requests
from bs4 import BeautifulSoup
# Initialize Selenium WebDriver
driver = webdriver.Chrome() # Ensure you have the ChromeDriver installed
driver.get("https://www.scrapingcourse.com/login")
# Perform login using Selenium (adjust selectors as needed)
email_input = driver.find_element(By.NAME, "email")
email_input.send_keys("[email protected]")
password_input = driver.find_element(By.NAME, "password")
password_input.send_keys("password")
password_input.send_keys(Keys.RETURN)
# Allow some time for login to process
time.sleep(5)
# Extract cookies from Selenium and transfer to requests session
session = requests.Session()
for cookie in driver.get_cookies():
session.cookies.set(cookie['name'], cookie['value'])
# Keep the browser open instead of quitting
input("Press Enter to continue after verifying the page is loaded...")
# Use the requests session to access a protected page
protected_page_url = "https://www.scrapingcourse.com/dashboard"
response = session.get(protected_page_url)
# Parse the response using BeautifulSoup
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
page_title = soup.title.string if soup.title else "No title found"
print(f"Page title: {page_title}")
else:
print(f"Failed to access the protected page: {response.status_code}")
print(response.text) # For debugging
This will be useful for you to confirm that the code is functioning correctly and logging into the correct page.
Conclusion
Scraping websites with login pages is fairly straightforward. Understanding the login page parameters would help explain how the website handles login requests. Most websites follow a similar mechanism with slightly different parameters, making the code you write for different websites quite easy to alter depending on the website of choice.
Handling sessions and managing cookies is a vital part of the code as without them, the session could time out or be made null, resulting in a possible IP ban. We have written articles detailing how to implement a CAPTCHA bypass tool as well as code to enter in a proxy within your script which would have added procedures for your scraping practices. With all of these tools blended together and functioning correctly, there should be no trouble for scraping websites with login pages with Python.