There are many reasons why someone would want to scrape YouTube videos. YouTube is an excellent data source for video performance, content trends, and audience engagement. Combing through the hundreds of hours a minute of content will be daunting and time consuming. With millions of hours of video content being uploaded monthly, manually analyzing trends is impractical, making automation a necessary solution. When you scrape YouTube videos, you can extract important details such as the title, duration, resolution, and upload date for deeper analysis.
With a script that can scrape YouTube videos, you will save countless hours and gather all the information you need to understand your target demographic and have an outlook of what is trending and why. While you can use the official YouTube API to scrape YouTube videos, the API is limited in its potential. This article will detail how you can use Python to extract videos and their metadata.
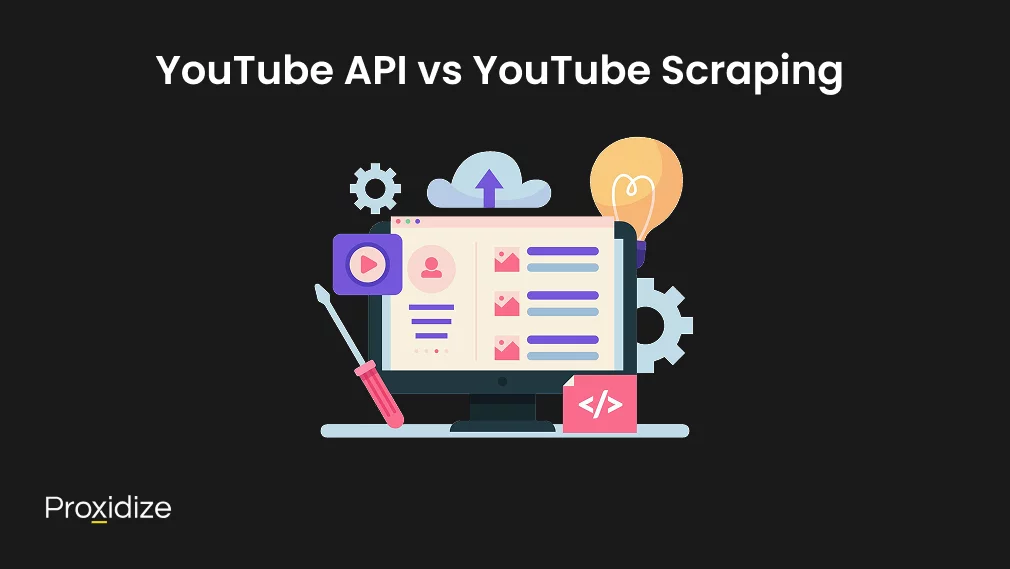
YouTube API vs YouTube Scraping
YouTube does have an official way to get data from its platform called the YouTube Data API. It scrapes information about videos, playlists, and content creators. There are many reasons why someone would choose to scrape YouTube videos themself rather than relying on this API. For starters, you can control what data you need to collect, if you decide to just scrape the titles and views, you can do that with your own scraper as the API has predefined data sets. When you scrape YouTube videos, you gain access to additional metadata and insights that may not be available through the API. Finally, YouTube APIs are subject to rate limiting as it determines the frequency and volume of requests you can make. By writing your own scraper, you can set your own limits and scrape YouTube videos freely without feeling limited by time or resources. While scraping YouTube offers more flexibility than the YouTube API, it’s important to review the platform’s terms of service to understand any restrictions.
The data that you can scrape from YouTube includes the video title, video description, views, likes, duration, publication date, name of the channel, user description, number of videos, and even the comments and related videos. If you want to scrape video thumbnails, you can extract the URL from the metadata and download the image.
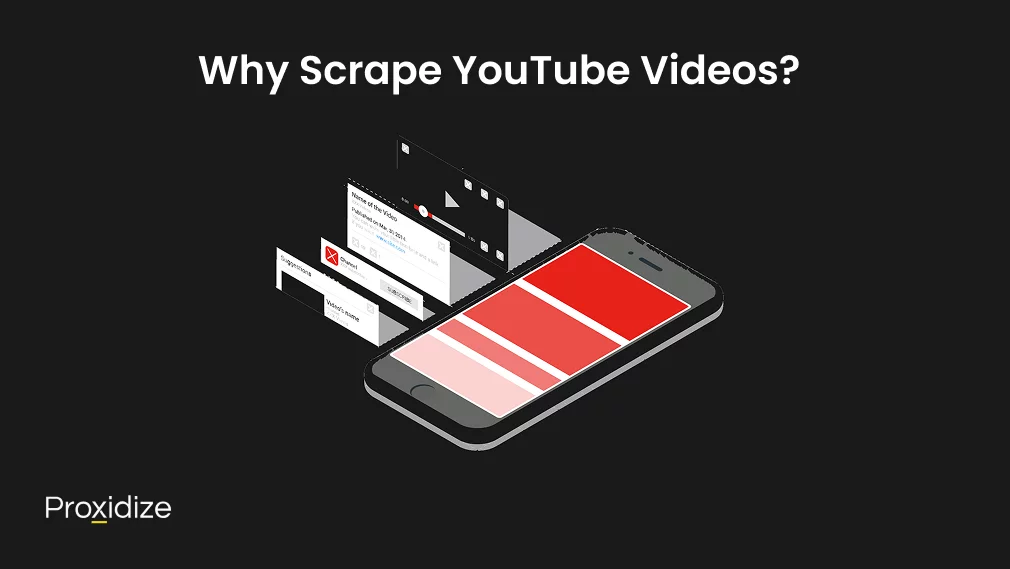
Why Scrape YouTube Videos?
There are many reasons as to why you should scrape YouTube videos. You could utilize YouTube for sentimental analysis to learn what people are saying about your business. You could gather valuable insights from text data. Many people use social media to express their thoughts on a brand’s products and services and would be transparent about their opinions, giving you the chance to get a full honest thought of your business practices. Details such as likes and comments are a great way to get insights about your customers. Understanding their preferences can help you improve and customize your products and services based on their insights. When you scrape YouTube videos, brands can analyze target audience behaviors, track trending topics, and determine which content generates the highest engagement. Companies track popular product review videos to gauge consumer sentiment and understand which products are gaining the most traction.
Additionally, you can expand your customer base by using YouTube data. One of the most effective forms of lead generation is through referral marketing also known as word of mouth. People tend to be more trustworthy of a recommendation by a friend or coworker than trusting advertisements. Engagement with customers on social media channels and resolving any complaints they might have is critical to strengthening the customer relationship. When you scrape YouTube videos, you can be informed of any negative comments stated about your business or product and do some damage control before it gets out of hand. Analyzing metrics including likes on video content and comments provides insight into audience approval of a particular video or brand.
YouTube’s algorithm ranks videos based on views. Understanding what works best for your specific audience is necessary for increasing your online presence on YouTube. Your company channel will most likely have a variety of video ideas. The YouTube Studio will help you monitor and analyze their performance which, while great, does not help you rank high on YouTube’s search results. Understanding what your competitors are doing can give you an understanding of what their strategy is. Certain factors such as trending topics and keywords can be the difference between ranking high or not ranking at all.
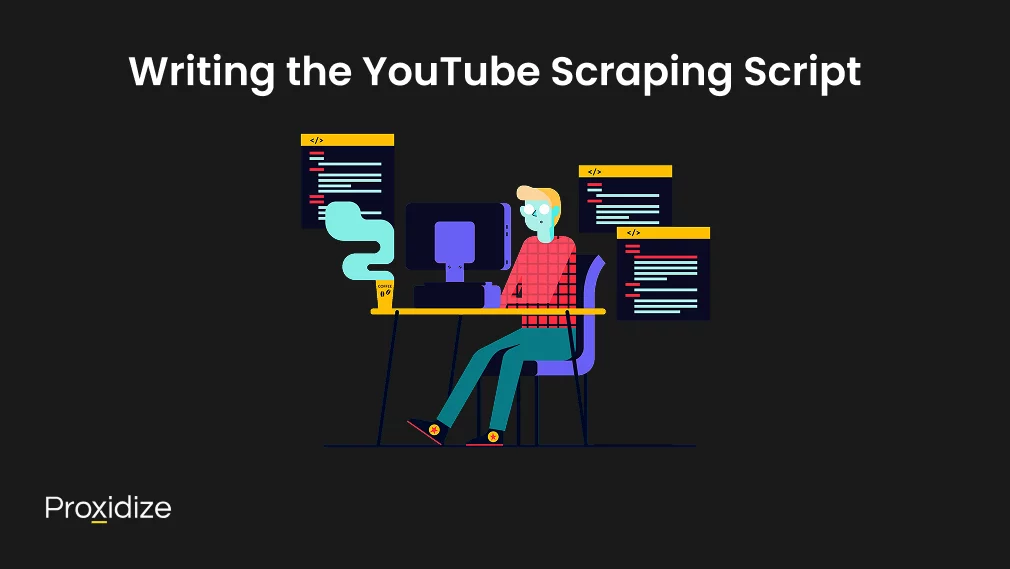
Writing the YouTube Scraping Script
With all that in mind, it is time to understand how to write the script. If you have written a scraping script before, then this process should be simple and straightforward. If this is your first attempt at writing a scraping script, do not worry. Each step will be explained in detail and each action will be justified.
Setting Up the Environment
We will present a complete code script in Python so the first step is to ensure that you have the latest version of Python installed. As of the writing of this article, the latest version of Python is 3.13.1. Once you have confirmed that you have the latest version of Python, open up your IDE and install the necessary libraries.
For this script, you will need the following essential libraries:
- Yt-dlp which will be necessary to scrape YouTube videos
- Requests, the simple HTTP library for Python
- BeautifulSoup4 for parsing HTML and extracting the needed data
The first thing you need to do is to create a new directory which will keep everything organized and easier to navigate through. This can be done by entering the following command in your terminal:
mkdir youtube-scraper
cd youtube-scraper
Next, you will need to install the necessary libraries. As we mentioned previously, these libraries are Requests, BeautifulSoup, and yt-dlp. Enter the following command in your terminal:
pip install yt-dlp requests beautifulsoup4
With these libraries installed, your environment should be ready to go. Now, we can start writing the script to scrape YouTube videos. We will cover how to scrape YouTube videos and then tackle how to scrape the information such as the titles, views, and comments.
Code to Scrape YouTube Videos
Before we move any further, it is important to note that most YouTube videos and the information included in them might be protected by copyright law, intellectual property law, or other similar rights. Before you start to scrape YouTube videos, it is strongly advised that you check if the video you intend to scrape falls under any such protected right.
To scrape YouTube videos, you will be using the yt-dlp library which is a popular library for downloading videos. For this example, we will be using this video from Proxidize’s own YouTube channel. To scrape the video, you will need to import the library and then use the download() method. Here is a sample script:
from yt_dlp import YoutubeDL
video_url = "https://www.youtube.com/watch?v=M-hAQ5nkSMs&pp=ygUJcHJveGlkaXpl"
opts = dict()
with YoutubeDL(opts) as yt:
yt.download([video_url])
After running the script, you may have found this error message:
WARNING: ffmpeg not found. The downloaded format may not be the best available. Installing ffmpeg is strongly recommended.
The reason this message pops up relates to how YouTube videos tend to have separate audio and video files to them. ffmpeg works with yt-dlp to merge the files together. Without it, there is a chance you will either get a video with no audio or a very low-quality video. This is an easy fix. All you need to do is download ffmpeg through their website.
If you wish to use this script to scrape YouTube videos, all you would need to do is replace the video_url
with the URL of the video of your choice. Remember to keep the video link within the semicolons. Once you run the script, the scraped YouTube video will be in the same folder as your .py
file.
Scraping YouTube Video Data
Now that you have the video, you have an easier way to watch the specific content you want to analyze. What if you wanted the video’s metadata? Well, that requires a whole different script but will still use the same prerequisites. You can use the extract_info
method with the download=False
parameter so you will not get the video download file again and will just get the needed information.
from yt_dlp import YoutubeDL
video_url = "https://www.youtube.com/watch?v=M-hAQ5nkSMs"
opts = dict()
with YoutubeDL(opts) as yt:
info = yt.extract_info(video_url, download=False)
video_title = info.get("title")
width = info.get("width")
height = info.get("height")
language = info.get("language")
channel = info.get("channel")
likes = info.get("like_count")
data = {
"URL": video_url,
"Title": video_title,
"Width": width,
"Height": height,
"Language": language,
"Channel": channel,
"Likes": likes
}
print(data)
This script will print out the video’s URL, its title, its resolution, and its language. If you plan to scrape YouTube videos with this script, remember to change the URL to the video you wish to gather the information from.
How to Scrape YouTube Comments
If you also want to scrape YouTube video comments, you will need to add the additional option getcomments
when using yt-dlp. Once you set getcomments
to True, the extract_info()
method will then gather all the comment threads with all the information attached to them. Your script will look like this:
from yt_dlp import YoutubeDL
from pprint import pprint
video_url = "https://www.youtube.com/watch?v=M-hAQ5nkSMs"
opts = {
"getcomments": True
}
with YoutubeDL(opts) as yt:
info = yt.extract_info(video_url, download=False)
comments = info["comments"]
thread_count = info["comment_count"]
print("Number of threads: {}".format(thread_count))
pprint(comments)
How to Scrape YouTube Channel Information
To scrape the YouTube channel information, things start to get a bit trickier. Scraping this information requires you to utilize a proxy server as without one, your script will either come up empty or you will get an error message stating that the attempt was blocked by YouTube’s anti-bot protection measure. Below, we have provided a script that will help you scrape a YouTube channel’s about page by using Proxidize’s rotating mobile proxies.
import requests
from bs4 import BeautifulSoup
import time
import random
# Replace with your Proxidize mobile proxy IP and port
PROXY_IP = "YOUR_PROXIDIZE_IP"
PROXY_PORT = "YOUR_PROXIDIZE_PORT"
PROXY_USERNAME = "YOUR_USERNAME" # If authentication is required
PROXY_PASSWORD = "YOUR_PASSWORD" # If authentication is required
# Proxy configuration
proxy_url = f"http://{PROXY_USERNAME}:{PROXY_PASSWORD}@{PROXY_IP}:{PROXY_PORT}"
proxies = {"http": proxy_url, "https": proxy_url}
# Headers to mimic a real browser
HEADERS = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/119.0.0.0 Safari/537.36",
"Accept-Language": "en-US,en;q=0.9",
}
# YouTube channel "About" page URL
channel_url = "https://www.youtube.com/@proxidize/about"
def get_channel_info():
try:
response = requests.get(channel_url, headers=HEADERS, proxies=proxies, timeout=10)
if response.status_code == 200:
soup = BeautifulSoup(response.text, "html.parser")
# Extract channel name and description
channel_name = soup.find("yt-formatted-string", {"id": "text", "class": "style-scope ytd-channel-name"})
channel_desc = soup.find("div", {"id": "wrapper", "class": "style-scope ytd-channel-tagline-renderer"})
return {
"channel_name": channel_name.text.strip() if channel_name else "Not Found",
"channel_desc": channel_desc.text.strip() if channel_desc else "Not Found",
}
else:
print(f"Failed to fetch page. Status Code: {response.status_code}")
return None
except Exception as e:
print(f"Error: {e}")
return None
# Rotating Proxies: Change IP after each request
def rotate_ip():
print("Rotating IP...")
time.sleep(random.randint(5, 10)) # Simulate human behavior
# Proxidize allows manual IP refresh (if needed, trigger it via API)
if __name__ == "__main__":
for _ in range(3): # Rotate 3 times
info = get_channel_info()
if info:
print(info)
rotate_ip()
This script will use a mobile proxy to access YouTube anonymously and fetch the YouTube channel’s name and description from the about page. It will also rotate the proxy after each request which will prevent any blocks and lessen your chances of getting an IP ban. It will also mimic a real browser by using headings which will allow you to run the script multiple times without getting blocked.
How to Scrape YouTube Search Results
Using a mobile proxy you can scrape the YouTube search results page. However, if you wish to do this, you will need to incorporate Selenium to bypass bot detection and render the JavaScript which Request and BS4 cannot do alone. The script below presents how you can scrape the YouTube search keyword “mobile proxies”.
import time
import requests
import undetected_chromedriver as uc
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
from bs4 import BeautifulSoup
# Proxidize API credentials
PROXIDIZE_API_KEY = "YOUR_PROXIDIZE_API_KEY"
PROXIDIZE_PROXY_URL = "YOUR_PROXIDIZE_PROXY_URL" # Format: http://USER:PASS@IP:PORT
# YouTube search URL for "mobile proxies"
search_query = "mobile proxies"
search_url = f"https://www.youtube.com/results?search_query={search_query.replace(' ', '+')}"
# Function to refresh Proxidize IP
def refresh_proxy():
response = requests.get(f"https://api.proxidize.com/v1/proxies/rotate?api_key={PROXIDIZE_API_KEY}")
if response.status_code == 200:
print("IP rotated successfully")
else:
print("Failed to rotate IP")
# Set up Selenium with Proxidize mobile proxy
def get_search_results():
options = uc.ChromeOptions()
options.add_argument(f"--proxy-server={PROXIDIZE_PROXY_URL}") # Use Proxidize proxy
options.add_argument("--headless") # Run in headless mode
options.add_argument("--disable-blink-features=AutomationControlled") # Hide Selenium
driver = uc.Chrome(options=options)
try:
driver.get(search_url)
time.sleep(5) # Allow page to load
# Get page source and parse it with BeautifulSoup
soup = BeautifulSoup(driver.page_source, "html.parser")
results = []
for video in soup.select("ytd-video-renderer"):
title = video.select_one("#video-title").text.strip()
link = "https://www.youtube.com" + video.select_one("#video-title")["href"]
views = video.select_one("#metadata-line span").text.strip() if video.select_one("#metadata-line span") else "N/A"
results.append({"title": title, "link": link, "views": views})
return results
finally:
driver.quit()
if __name__ == "__main__":
refresh_proxy() # Rotate IP before scraping
search_results = get_search_results()
if search_results:
for idx, video in enumerate(search_results[:10]): # Print first 10 results
print(f"{idx+1}. {video['title']} - {video['views']}\n {video['link']}\n")
Full Script to Scrape YouTube Videos, Including Metadata
If you want to scrape everything from the YouTube video to the comments and the channel, its description, and a YouTube search result, we have you covered. This script will take care of it all. All you would need to do is enter your proxy information and change the URLs to whichever page and/or account you wish to scrape. As an added bonus, this script will save all the information into a JSON file. When scraping at scale, using automated CAPTCHA-solving services can help bypass YouTube’s bot detection mechanisms.
import time
import requests
import undetected_chromedriver as uc
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
from bs4 import BeautifulSoup
# Proxidize API credentials
PROXIDIZE_API_KEY = "YOUR_PROXIDIZE_API_KEY"
PROXIDIZE_PROXY_URL = "YOUR_PROXIDIZE_PROXY_URL" # Format: http://USER:PASS@IP:PORT
# YouTube search URL for "mobile proxies"
search_query = "mobile proxies"
search_url = f"https://www.youtube.com/results?search_query={search_query.replace(' ', '+')}"
# Function to refresh Proxidize IP
def refresh_proxy():
response = requests.get(f"https://api.proxidize.com/v1/proxies/rotate?api_key={PROXIDIZE_API_KEY}")
if response.status_code == 200:
print("IP rotated successfully")
else:
print("Failed to rotate IP")
# Set up Selenium with Proxidize mobile proxy
def get_search_results():
options = uc.ChromeOptions()
options.add_argument(f"--proxy-server={PROXIDIZE_PROXY_URL}") # Use Proxidize proxy
options.add_argument("--headless") # Run in headless mode
options.add_argument("--disable-blink-features=AutomationControlled") # Hide Selenium
driver = uc.Chrome(options=options)
try:
driver.get(search_url)
time.sleep(5) # Allow page to load
# Get page source and parse it with BeautifulSoup
soup = BeautifulSoup(driver.page_source, "html.parser")
results = []
for video in soup.select("ytd-video-renderer"):
title = video.select_one("#video-title").text.strip()
link = "https://www.youtube.com" + video.select_one("#video-title")["href"]
views = video.select_one("#metadata-line span").text.strip() if video.select_one("#metadata-line span") else "N/A"
results.append({"title": title, "link": link, "views": views})
return results
finally:
driver.quit()
if __name__ == "__main__":
refresh_proxy() # Rotate IP before scraping
search_results = get_search_results()
if search_results:
for idx, video in enumerate(search_results[:10]): # Print first 10 results
print(f"{idx+1}. {video['title']} - {video['views']}\n {video['link']}\n")
Error Handling
Before you scrape YouTube videos and their metadata, there are several challenges to consider, such as request limits, dynamic content loading, and bot detection measures. YouTube actively monitors automated traffic, which can result in 403 Forbidden or 429 Too Many Requests errors if scraping requests are too frequent. To avoid this, implementing IP rotation, adjusting request intervals, and using proxies can help maintain uninterrupted scraping. Since YouTube dynamically loads elements like views and comments, using tools that can render JavaScript, such as Selenium, may be necessary. By applying these techniques, you can improve the reliability and efficiency of your YouTube scraping process while minimizing disruptions.
Conclusion
When you scrape YouTube videos and other relevant data, you are introduced to valuable insights into content performance and audience engagement. By using Python and its many libraries as well as adhering to guidelines, you can effectively scrape YouTube videos.
Key takeaways:
- Scrape YouTube videos to extract data beyond the limitations of the YouTube Data API.
- Python libraries like yt-dlp, requests, and BeautifulSoup make it easy to scrape YouTube videos for metadata, comments, and search results.
- Installing ffmpeg ensures high-quality video downloads when using yt-dlp to scrape YouTube videos, as it merges separate audio and video streams.
- Using proxies and rotating IPs while attempting to scrape YouTube videos helps avoid detection and prevents rate limiting.
However, it’s crucial to remain mindful of legal considerations and platform policies when attempting to scrape YouTube videos to ensure responsible and lawful data collection. Through using proxies, request delays, and error handling, you can optimize the process and prevent detection while scraping.
Frequently Asked Questions
What is the best tool for market researchers analyzing YouTube data?
A powerful tool for market researchers should include features like proxy support, search result extraction, and audience sentiment analysis.
How do I avoid getting blocked while deciding to scrape YouTube videos?
Utilizing IP rotation, mobile proxies, and adjusting your scraping speed should help avoid detection.
Can I store my scraped data in Google Drive or Google Sheets?
Yes, you can export your data to a Google Sheet or save JSON files in Google Drive for easy access.
What are common issues to keep in mind when choosing to scrape YouTube videos?
Common issues include IP bans, CAPTCHA challenges, and dynamic content loading that prevents data extraction. However, they can be bypassed by using a proxy, implementing a CAPTCHA solver, and using Selenium to handle dynamic content.