Choosing between Puppeteer vs Selenium should be a straightforward concept but there are various things to keep in mind before blindly picking one over the other. They are both well-known open-source tools that are mainly used for browser automation and testing. While Puppeteer is a newer addition to the programming world, it has gained appreciation from developers due to its useful features and great performance. Selenium on the other hand has existed since 2004 and remains an industry leader in automation and offering support for multiple programming languages and platforms. This article aims to compare Puppeteer vs Selenium by looking at their installation and general differences before answering the question of Puppeteer vs Selenium.
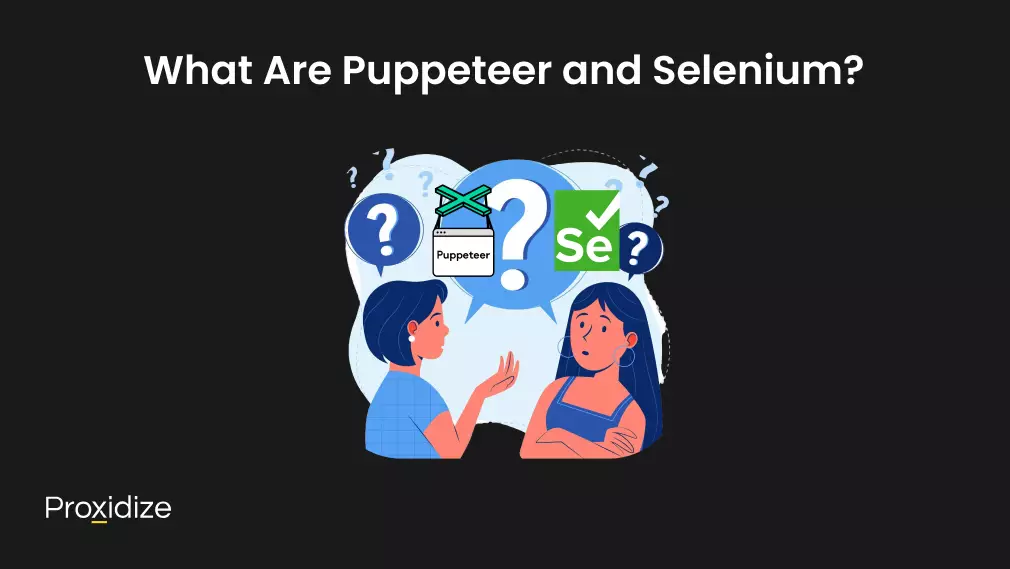
What Are Puppeteer and Selenium?
Before we go further into the differences of Puppeteer vs Selenium, it is important to get some pretext of what Puppeteer and Selenium are and what they are most commonly used for. This might build the idea in your mind on which one sounds better for your use case.
Puppeteer
Puppeteer is a Node.js library that is mainly used for creating an automated testing environment, It was developed by Google as a way to provide a high-level API to control Chrome and Chromium over the DevTools Protocol. Puppeteer focuses on offering a specific set of control structures as it only supports JavaScript and serves as a remote control library for Chrome. Puppeteer is a popular choice amongst both developers and beginners.
Puppeteer is used for a variety of tasks such as testing Chrome extensions, taking screenshots and generating PDFs of web pages, performing tests on the latest versions of Chromium, automating manual testing processes, and web scraping.
When it comes to its advantages, Puppeteer is beginner-friendly, has an event-driven architecture, supports Chrome DevTools Protocol (CDP) and Remote Debugging Protocol (RDP), can generate browser automation scripts directly from the Chrome DevTools recorder, has faster execution speeds, offers a headless mode which allows tests to run without a visible browser window, and includes built-in capabilities for collecting screenshots and creating PDF files.
As for its limitations, Puppeteer only supports Chrome and Firefox, limiting anyone who prefers to use other browsers. Puppeteer also only focuses on JavaScript with some unofficial ports for Python such as Pyppeteer.
Selenium
Selenium is a testing library that supports almost all the major web browsers including Chrome, Chromium, Firefox, Safari, Opera, and Microsoft Edge. Selenium can also be written using JavaScript, Ruby, C#, Java, and Python, making it more flexible for anyone who has not learned how to write in JavaScript. Selenium has an IDE which includes Selenium WebDriver, Selenium IDE, and Selenium Grid which extend the library’s capabilities and allow users to satisfy different testing needs.
Selenium is commonly used for web performance testing, application testing, automation testing, performance testing, and web scraping. As for its advantages, Selenium is open source and freely available, integrated with CI and Agile, supports cross-browser use, has dedicated community support, and has been around since 2004 meaning it had more time to gain a reputation as a solid and stable choice.
Its downsides lie in its steep learning curve, lack of support for built-in image comparison, limited support for DevTools Protocol, dependence on an extension for automation script generation, and complex setup due to its different drivers for each browser.
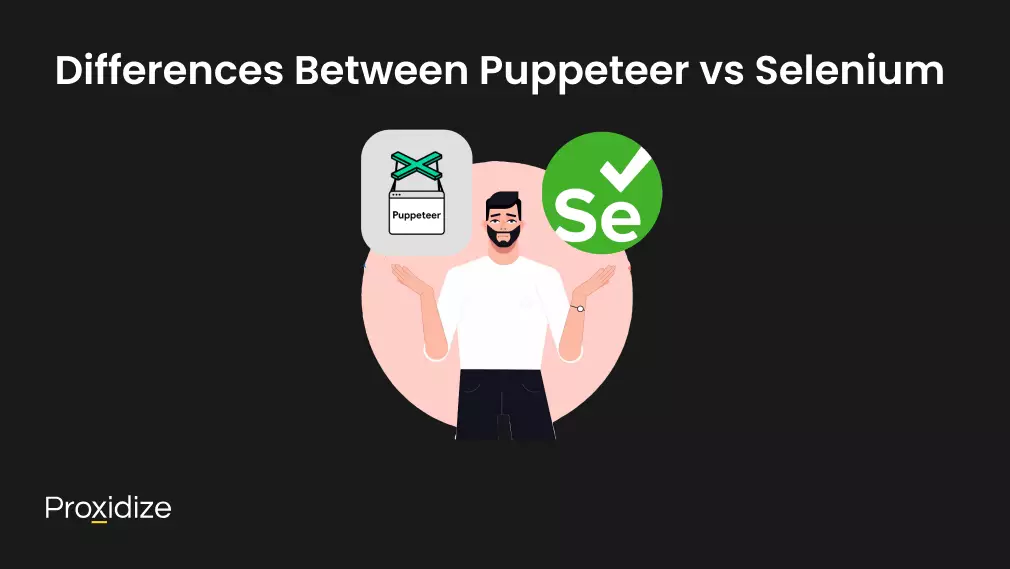
Differences Between Puppeteer vs Selenium
Now that we have explained both tools, it is imperative to compare Puppeteer vs Selenium before exploring closely how they differ in terms of setting up the environment and how their code scripts differ.
Browser Support
Puppeteer is mainly used to work with Chromium-based browsers and Firefox. This gives direct access to advanced Chromium browser features and APIs. With this integration, Puppeteer is highly compatible with web standards which results in consistent behavior of test scripts across different environments. However, its limited browser environments make it challenging for anyone more accustomed to non-Chrome-based browsers or Firefox.
Selenium provides support for nearly all browsers including Chrome, Firefox, Safari, and Edge. This ensures more coverage and more comprehensive testing scenarios. However, this can introduce challenges because each browser interprets and displays content differently, meaning achieving consistent synchronization across different browsers requires more time.
Language Support
Puppeteer was designed exclusively for Node.js and JavaScript environments. It can also run JS within web pages, making it valuable for effectively interacting with dynamic web pages and pre-rending content for JavaScript-heavy websites. Selenium supports multiple different programming languages including Java, Python, C#, Ruby, and JavaScript. This broadens its appeal across various developer communities and makes it easier to integrate into different developmental testing environments.
Use Cases
Puppeteer vs Selenium is a tough choice as both tools are widely used for web scraping. Puppeteer is suited for tasks that need deep integration with Chromium’s functionality. This includes generating screenshots, PDFs, crawling and scraping dynamic content, and rendering SEO-friendly content for JavaScript-heavy websites. Its ability to execute JS on the page makes it a perfect choice for extracting data from website applications that rely heavily on client-side scripts.
Selenium is wonderful for situations where cross-browser compatibility is necessary. It is a preferred tool for scraping from websites that need to be tested across different browsers. Its WebDriver protocol guarantees realistic user interactions which makes it valuable for automating data collection from interactive web pages. This becomes useful when scraping user-generated content, monitoring changes on real estate or e-commerce websites, and gathering extensive datasets from different applications for market analysis or research.
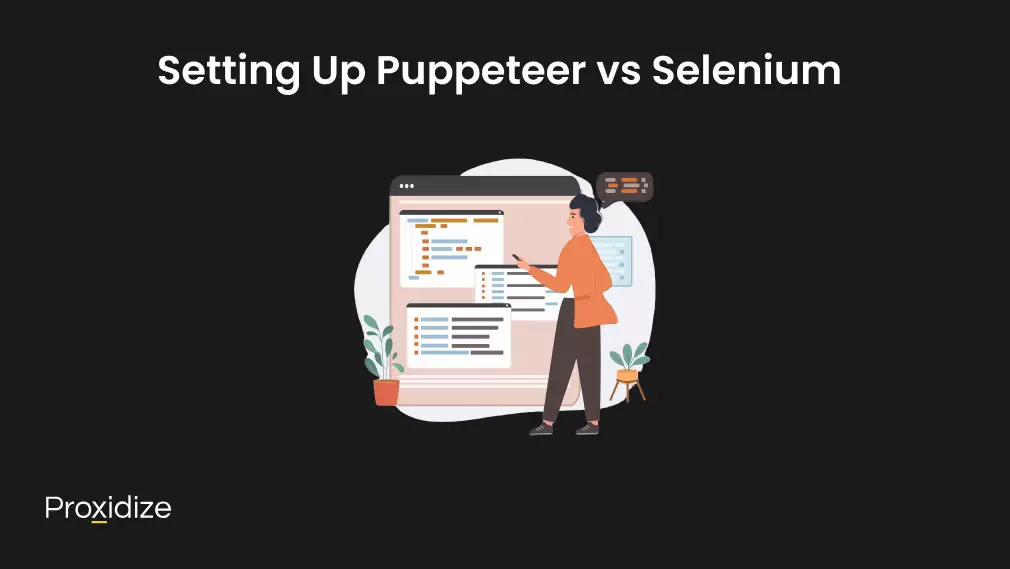
Setting Up Puppeteer vs Selenium
For this article, we will be comparing Puppeteer vs Selenium in terms of how they are written for web scraping. We will be using the website http://quotes.toscrape.com as our testing ground. We have used this website many times before including in our web scraping with Ruby tutorial.
Installation
When looking at Puppeteer vs Selenium’s installation, both have a simple and straightforward path. The only difference is the prerequisite libraries. Puppeteer simply needs the npm command while Selenium requires following language-specific instructions. For the sake of simplicity and equality, we will present Selenium’s installation and pathways in JavaScript.
Puppeteer
npm install puppeteer
Selenium
npm install selenium-webdriver
npm install chromedriver
Browser Control and Web Scraping
Puppeteer vs Selenium allows for programmatic web browser control. This allows you to scrape dynamic content from a website. In this section, we will look at the key code differences in launching a Headless Chrome instance, navigating it to a specific page, waiting for the dynamic content to load, and scraping the page. As mentioned above, we will be using the Quotes To Scrape website which is a dynamic web page where all the quotes are loaded dynamically through the relevant JavaScript file. The file renders quotes in <div> elements that all have a quote class.
Dependencies and Target Setting
Puppeteer
const puppeteer = require('puppeteer');
const url = 'http://quotes.toscrape.com/js/';
Selenium
const { Builder, By, Key, until } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
const url = 'http://quotes.toscrape.com/js/';
Selenium’s support of a range of browsers requires importing specific browser drivers along with WebDriver. Chrome drive is implicit with Puppeteer.
Launching Headless Chrome and Navigating to URL
Puppeteer
const headlessBrowser = await puppeteer.launch({ headless: true });
const newTab = await headlessBrowser.newPage();
await newTab.goto(url);
Selenium
let driver = await new Builder().forBrowser('chrome') .setChromeOptions(new chrome.Options().headless()).build();
await driver.get(url);
Puppeteer uses the await launch() method to launch the browser and the newPage() method to create a new browser tab. The goto() method can now navigate the tab to any URL. Selenium uses the Builder() constructor to build a new Builder followed by specific options. The build() method at the end is there to create and return a new instance of the WebDriver session.
Waiting for Content to Load
When waiting for JavaScript content to load while using Puppeteer vs Selenium, the code tends to wait for JS to load a <div> element with the quote class
Puppeteer
await newTab.waitForSelector('.quote');
Selenium
await driver.wait(until.elementLocated(By.className('quote')));
Puppeteer uses the waitForSelector() method while Selenium uses the simpler wait() method along with (until) to wait for a specific element to load.
Scraping the Content
Puppeteer uses querySelectorAll() to select and return a list of all the matching elements. Selenium uses the findElements() method to extract the relevant elements matching the By selectors.
Puppeteer
let quotes = await newTab.evaluate(() => {
let allQuoteDivs = document.querySelectorAll(".quote");
let quotesString= "";
allQuoteDivs.forEach((quote) => {
let quoteText = quote.querySelector(".text").innerHTML;
quotesString += `${quoteText} \n`;
});
return quotesString;
});
console.log(quotes);
Selenium
let quotes = await driver.findElements(By.className('quote'));
let quotesString = "";
for (let quote of quotes) {
let quoteText = await quote.findElement(By.className('text')). getText();
quotesString += `${quoteText} \n`;
}
console.log(quotesString);
The evaluate() method in the Puppeteer code allows executing a function in the current tab or page context. You can access and manipulate the elements in the DOM of the current tab and then return the value as a result.
Closing the Browser
Puppeteer has the close() method to close the browser while Selenium offers the quit() method.
Puppeteer
headlessBrowser.close();
Selenium
await driver.quit();
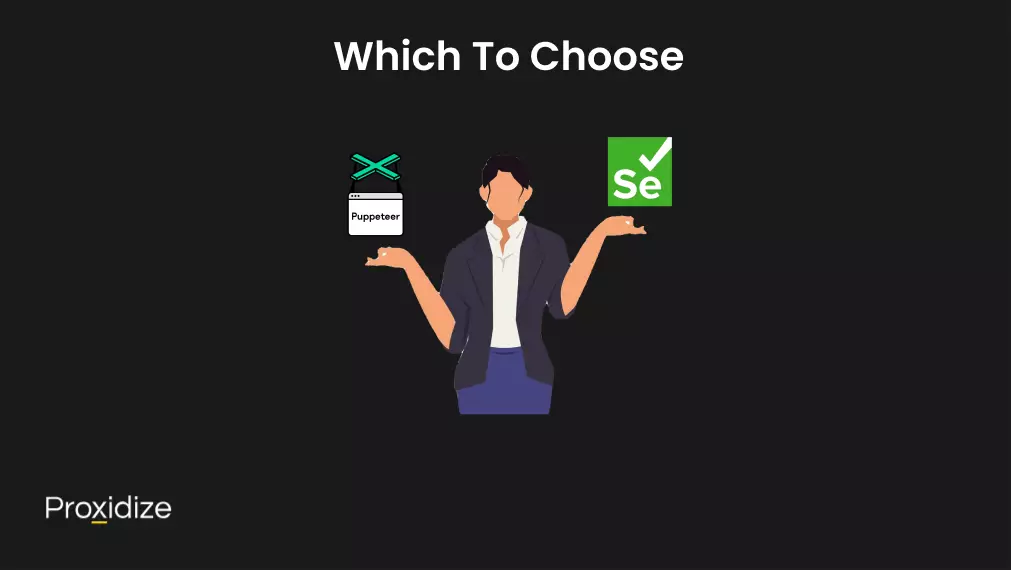
Which To Choose
With everything laid out, you might feel a bit overwhelmed with all the information provided. As such, we will give you an easy-to-digest guide to help you finally make your decision.
Choosing Puppeteer vs Selenium is difficult as both are powerful tools with exceptional capabilities for testing automation. Their differences depend on quite a few factors that range from comfort to familiarity.
If you or your team work with Chrome or Firefox, then Puppeteer is the perfect choice. Its high-level API will give you unparalleled control over the browser and the speeds and focus offered by Puppeteer will ensure you achieve efficiency in setting up tests. Considering Puppeteer is mostly used for web automation rather than testing, it will be more suitable for web crawling and scraping.
However, if you are more akin to non-Chromium-based browsers and non-JavaScript coding, Selenium might be right up your alley. Selenium WebDriver offers cross-browser support, giving you the ability to interact with any browser directly.
Browser choices and programming language should not be the only factors to consider in Puppeteer vs Selenium as there are other functionalities such as record and playback for testing web applications that could be the deciding choice. If that is something that matters to you or your team, then Selenium would be a better choice than Puppeteer. Selenium’s code can be re-used and is loaded with packages and test suites. It is also considered to be the best tool for automation testing.
To truly narrow it down, if you need cross-browser testing or work with multiple languages, Selenium is the go-to as its long history and large community provide many resources for learning and support. If your focus is purely on web scraping, generating PDFs, or Chrome-based testing, then Puppeteer is the better choice.
Avoiding Blocks
While Puppeteer vs Selenium offers unique scraping features, they do tend to leak bot-like attributes such as the HeadlessChrome User Agent and missing or suspicious fingerprints. These limits make them risky to use without getting detected by the website you intend to scrape. To circumvent these issues, it is recommended to use an antidetect browser, a headless browser, or a virtual machine. Similarly, you could use a proxy server to hide your IP address. With a mobile proxy, you can introduce an intermediary between your server and your device, keeping your identity hidden and your actions secure as you scrape from websites that have anti-bot measures in place.
Conclusion
When deciding between Puppeteer vs Selenium, your choice should come down to your specific project requirements. Puppeteer is seamless with Chromium and makes it ideal for tasks that rely heavily on Chrome or Firefox such as scraping and PDF generation. Selenium’s broad browser and language support along with its long-standing reputation makes it a strong contender for cross-browser testing and more diverse automation scenarios.
Key takeaways:
- Puppeteer vs Selenium are both excellent tools for browser automation but Puppeteer focuses on JS and Chrome while Selenium offers cross-browser and multi-language support.
- If you need high-speed browser automation or advanced Chromium features, pick Puppeteer.
- Selenium is a better choice for teams that need cross-browser testing or are more comfortable and capable of working with different programming languages than just JavaScript.
- Puppeteer vs Selenium differ in how they are installed. Puppeteer is more streamlined but is limited to just Chrome browsers while Selenium is flexible but often more complex with additional prompts to add.
- While both Puppeteer vs Selenium are great for web scraping, Puppeteer’s integration with Chrome makes it more efficient for scraping JS-heavy websites.
Weighing the strengths and limitations of Puppeteer vs Selenium ensures that you pick the right tool to optimize your workflow and ensure a successful project with little to no infractions.